@ -1,10 +1,11 @@ |
|||||||
{ |
{ |
||||||
"baseUrl": "http://localhost:3000", |
"baseUrl": "http://localhost:3000/", |
||||||
"defaultCommandTimeout": 13000, |
"defaultCommandTimeout": 13000, |
||||||
"pageLoadTimeout": 600000, |
"pageLoadTimeout": 600000, |
||||||
"viewportWidth": 1980, |
"viewportWidth": 1980, |
||||||
"viewportHeight": 1000, |
"viewportHeight": 1000, |
||||||
"video": false, |
"video": false, |
||||||
"retries": 2, |
"retries": 2, |
||||||
"screenshotOnRunFailure": false |
"screenshotOnRunFailure": false, |
||||||
|
"numTestsKeptInMemory": 5 |
||||||
} |
} |
||||||
|
@ -0,0 +1,93 @@ |
|||||||
|
|
||||||
|
// Cypress test suite: project pre-configurations
|
||||||
|
//
|
||||||
|
|
||||||
|
import { loginPage, projectsPage } from "../../support/page_objects/navigation" |
||||||
|
import { mainPage } from "../../support/page_objects/mainpage" |
||||||
|
import { staticProjects, roles } from "../../support/page_objects/projectConstants" |
||||||
|
|
||||||
|
describe(`Project pre-configurations`, () => { |
||||||
|
|
||||||
|
it('Admin SignUp', ()=> { |
||||||
|
cy.waitForSpinners(); |
||||||
|
cy.signinOrSignup(roles.owner.credentials) |
||||||
|
cy.wait(2000) |
||||||
|
}) |
||||||
|
|
||||||
|
const createProject = (proj) => { |
||||||
|
it(`Create ${proj.basic.name} project`, () => { |
||||||
|
|
||||||
|
// click home button
|
||||||
|
mainPage.toolBarTopLeft(mainPage.HOME).click() |
||||||
|
// create requested project
|
||||||
|
projectsPage.createProject( proj.basic, proj.config ) |
||||||
|
})
|
||||||
|
} |
||||||
|
|
||||||
|
createProject(staticProjects.sampleREST) |
||||||
|
createProject(staticProjects.sampleGQL) |
||||||
|
createProject(staticProjects.externalREST) |
||||||
|
createProject(staticProjects.externalGQL) |
||||||
|
}) |
||||||
|
|
||||||
|
describe('Static user creations (different roles)', () => { |
||||||
|
|
||||||
|
beforeEach(()=> { |
||||||
|
loginPage.signIn(roles.owner.credentials) |
||||||
|
projectsPage.openProject(staticProjects.sampleREST.basic.name) |
||||||
|
}) |
||||||
|
|
||||||
|
const addUser = (user) => { |
||||||
|
it(`RoleType: ${user.name}`, () => { |
||||||
|
mainPage.addNewUserToProject(user.credentials, user.name) |
||||||
|
}) |
||||||
|
} |
||||||
|
|
||||||
|
addUser(roles.creator) |
||||||
|
addUser(roles.editor) |
||||||
|
addUser(roles.commenter) |
||||||
|
addUser(roles.viewer)
|
||||||
|
}) |
||||||
|
|
||||||
|
describe('Static users- add to other static projects', () => { |
||||||
|
|
||||||
|
const addUserToProject = (proj) => { |
||||||
|
it(`Add users to ${proj.basic.name}`, () => { |
||||||
|
loginPage.signIn(roles.owner.credentials) |
||||||
|
projectsPage.openProject(proj.basic.name) |
||||||
|
|
||||||
|
mainPage.addExistingUserToProject(roles.creator.credentials.username, roles.creator.name) |
||||||
|
mainPage.addExistingUserToProject(roles.editor.credentials.username, roles.editor.name) |
||||||
|
mainPage.addExistingUserToProject(roles.commenter.credentials.username, roles.commenter.name) |
||||||
|
mainPage.addExistingUserToProject(roles.viewer.credentials.username, roles.viewer.name) |
||||||
|
}) |
||||||
|
} |
||||||
|
|
||||||
|
addUserToProject(staticProjects.sampleGQL) |
||||||
|
addUserToProject(staticProjects.externalREST) |
||||||
|
addUserToProject(staticProjects.externalGQL) |
||||||
|
}) |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* @copyright Copyright (c) 2021, Xgene Cloud Ltd |
||||||
|
* |
||||||
|
* @author Raju Udava <sivadstala@gmail.com> |
||||||
|
* |
||||||
|
* @license GNU AGPL version 3 or any later version |
||||||
|
* |
||||||
|
* This program is free software: you can redistribute it and/or modify |
||||||
|
* it under the terms of the GNU Affero General Public License as |
||||||
|
* published by the Free Software Foundation, either version 3 of the |
||||||
|
* License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU Affero General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU Affero General Public License |
||||||
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||||
|
* |
||||||
|
*/ |
@ -0,0 +1,157 @@ |
|||||||
|
import { loginPage, projectsPage } from "../../support/page_objects/navigation" |
||||||
|
|
||||||
|
const shareViewWithPwd = (pwdCorrect, pwd) => { |
||||||
|
|
||||||
|
cy.get('.v-navigation-drawer__content > .container') |
||||||
|
.find('.v-list > .v-list-item') |
||||||
|
.contains('Share View') |
||||||
|
.click() |
||||||
|
|
||||||
|
// No password- unprotected link
|
||||||
|
if(''==pwd) { |
||||||
|
|
||||||
|
// copy link text, visit URL
|
||||||
|
cy.getActiveModal().find('.share-link-box') |
||||||
|
.contains('http', {timeout: 2000}) |
||||||
|
.then(($obj) => { |
||||||
|
|
||||||
|
let linkText = $obj.text() |
||||||
|
cy.log(linkText) |
||||||
|
cy.visit(linkText) |
||||||
|
|
||||||
|
// wait for share view page to load!
|
||||||
|
cy.wait(5000) |
||||||
|
cy.get('body').find('.v-dialog.v-dialog--active').should('not.exist') |
||||||
|
}) |
||||||
|
|
||||||
|
// password protected link
|
||||||
|
} else { |
||||||
|
|
||||||
|
// enable checkbox & feed pwd, save
|
||||||
|
cy.getActiveModal().find('[role="switch"][type="checkbox"]').click( {force: true} ) |
||||||
|
cy.getActiveModal().find('input[type="password"]').type('123456') |
||||||
|
cy.getActiveModal().find('button:contains("Save password")').click() |
||||||
|
|
||||||
|
// copy link text, visit URL
|
||||||
|
cy.getActiveModal().find('.share-link-box') |
||||||
|
.then(($obj) => { |
||||||
|
|
||||||
|
let linkText = $obj.text() |
||||||
|
cy.log(linkText) |
||||||
|
cy.visit(linkText) |
||||||
|
|
||||||
|
// wait for share view page to load!
|
||||||
|
cy.wait(5000) |
||||||
|
|
||||||
|
// feed password
|
||||||
|
cy.getActiveModal().find('input[type="password"]').type(pwd) |
||||||
|
cy.getActiveModal().find('button:contains("Unlock")').click() |
||||||
|
|
||||||
|
cy.wait(1000) |
||||||
|
|
||||||
|
// if pwd is incorrect, active modal requesting to feed in password again
|
||||||
|
// will remain
|
||||||
|
if(pwdCorrect) { |
||||||
|
cy.get('body').find('.v-dialog.v-dialog--active').should('not.exist') |
||||||
|
} |
||||||
|
else { |
||||||
|
cy.get('body').find('.v-dialog.v-dialog--active').should('exist') |
||||||
|
} |
||||||
|
})
|
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
// delete created views
|
||||||
|
//
|
||||||
|
const deleteCreatedViews = () => { |
||||||
|
cy.get('.v-navigation-drawer__content > .container') |
||||||
|
.find('.v-list > .v-list-item') |
||||||
|
.contains('Share View') |
||||||
|
.parent().find('button.mdi-dots-vertical').click() |
||||||
|
|
||||||
|
cy.getActiveMenu().find('.v-list-item').contains('Views List').click() |
||||||
|
|
||||||
|
cy.get(1000) |
||||||
|
|
||||||
|
cy.get('th:contains("View Link")').parent().parent() |
||||||
|
.next().find('tr').each((tableRow) => { |
||||||
|
cy.wrap(tableRow).find('button').last().click() |
||||||
|
cy.wait(1000) |
||||||
|
}) |
||||||
|
} |
||||||
|
|
||||||
|
const genTest = (type) => { |
||||||
|
|
||||||
|
describe(`${type.toUpperCase()} api - Clipboard access`, () => { |
||||||
|
|
||||||
|
let projectName |
||||||
|
|
||||||
|
// create project with default credentials to work with
|
||||||
|
//
|
||||||
|
before( () => { |
||||||
|
|
||||||
|
loginPage.signIn({ username: 'user@nocodb.com', password: 'Password123.' }) |
||||||
|
|
||||||
|
if(type == 'rest') |
||||||
|
projectName = projectsPage.createDefaulRestProject()
|
||||||
|
else |
||||||
|
projectName = projectsPage.createDefaultGraphQlProject() |
||||||
|
}) |
||||||
|
|
||||||
|
// Run once before test- create project (rest/graphql)
|
||||||
|
//
|
||||||
|
beforeEach(() => { |
||||||
|
loginPage.signIn({ username: 'user@nocodb.com', password: 'Password123.' }) |
||||||
|
projectsPage.openProject(projectName) |
||||||
|
|
||||||
|
cy.openTableTab('City'); |
||||||
|
}) |
||||||
|
|
||||||
|
it('Share view without password', () => { |
||||||
|
shareViewWithPwd(true, '') |
||||||
|
}) |
||||||
|
|
||||||
|
it('Share view with correct password', () => { |
||||||
|
shareViewWithPwd(true, '123456') |
||||||
|
}) |
||||||
|
|
||||||
|
it('Share view with incorrect password', () => { |
||||||
|
shareViewWithPwd(false, 'abc') |
||||||
|
}) |
||||||
|
|
||||||
|
it('Delete view', deleteCreatedViews ) |
||||||
|
|
||||||
|
// clean up
|
||||||
|
after( () => { |
||||||
|
loginPage.signIn({ username: 'user@nocodb.com', password: 'Password123.' }) |
||||||
|
projectsPage.deleteProject(projectName) |
||||||
|
}) |
||||||
|
}) |
||||||
|
} |
||||||
|
|
||||||
|
genTest('rest') |
||||||
|
genTest('graphql') |
||||||
|
|
||||||
|
/** |
||||||
|
* @copyright Copyright (c) 2021, Xgene Cloud Ltd |
||||||
|
* |
||||||
|
* @author Pranav C Balan <pranavxc@gmail.com> |
||||||
|
* @author Raju Udava <sivadstala@gmail.com> |
||||||
|
* |
||||||
|
* @license GNU AGPL version 3 or any later version |
||||||
|
* |
||||||
|
* This program is free software: you can redistribute it and/or modify |
||||||
|
* it under the terms of the GNU Affero General Public License as |
||||||
|
* published by the Free Software Foundation, either version 3 of the |
||||||
|
* License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU Affero General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU Affero General Public License |
||||||
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||||
|
* |
||||||
|
*/ |
@ -0,0 +1,65 @@ |
|||||||
|
|
||||||
|
export const defaultDbParams = { |
||||||
|
databaseType: 0, // MySQL
|
||||||
|
hostAddress: 'localhost', |
||||||
|
portNumber: '3306', |
||||||
|
username: 'root', |
||||||
|
password: 'password', |
||||||
|
databaseName: 'sakila' |
||||||
|
} |
||||||
|
|
||||||
|
// database
|
||||||
|
// validation details
|
||||||
|
// advSettings: left navigation bar (audit, metadata, auth, transient view modes)
|
||||||
|
// editSchema: create table, add/update/delete column
|
||||||
|
// editData: add/ update/ delete row, cell contents
|
||||||
|
// editComment: add comment
|
||||||
|
// shareView: right navigation bar (share options)
|
||||||
|
export const roles = { |
||||||
|
owner: { |
||||||
|
name: 'owner', |
||||||
|
credentials: { username: 'user@nocodb.com', password: 'Password123.' }, |
||||||
|
validations: { advSettings: true, editSchema: true, editData: true, editComment: true, shareView: true } |
||||||
|
}, |
||||||
|
creator: { |
||||||
|
name: 'creator', |
||||||
|
credentials: { username: 'creator@nocodb.com', password: 'Password123.' }, |
||||||
|
validations: { advSettings: true, editSchema: true, editData: true, editComment: true, shareView: true } |
||||||
|
}, |
||||||
|
editor: { |
||||||
|
name: 'editor', |
||||||
|
credentials: { username: 'editor@nocodb.com', password: 'Password123.' }, |
||||||
|
validations: { advSettings: false, editSchema: false, editData: true, editComment: true, shareView: false } |
||||||
|
}, |
||||||
|
commenter: { |
||||||
|
name: 'commenter', |
||||||
|
credentials: { username: 'commenter@nocodb.com', password: 'Password123.' }, |
||||||
|
validations: { advSettings: false, editSchema: false, editData: false, editComment: true, shareView: false } |
||||||
|
}, |
||||||
|
viewer: { |
||||||
|
name: 'viewer', |
||||||
|
credentials: { username: 'viewer@nocodb.com', password: 'Password123.' }, |
||||||
|
validations: { advSettings: false, editSchema: false, editData: false, editComment: false, shareView: false } |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
// default projects
|
||||||
|
//
|
||||||
|
export const staticProjects = { |
||||||
|
sampleREST: { |
||||||
|
basic: { dbType: 'none', apiType: 'REST', name: 'sampleREST' },
|
||||||
|
config: {} |
||||||
|
}, |
||||||
|
sampleGQL: { |
||||||
|
basic: { dbType: 'none', apiType: 'GQL', name: 'sampleGQL' },
|
||||||
|
config: {} |
||||||
|
}, |
||||||
|
externalREST: { |
||||||
|
basic: { dbType: 'external', apiType: 'REST', name: 'externalREST' },
|
||||||
|
config: defaultDbParams |
||||||
|
}, |
||||||
|
externalGQL: { |
||||||
|
basic: { dbType: 'external', apiType: 'GQL', name: 'externalGQL' },
|
||||||
|
config: defaultDbParams |
||||||
|
} |
||||||
|
} |
@ -1,27 +0,0 @@ |
|||||||
# Start |
|
||||||
|
|
||||||
# Social Media |
|
||||||
- Twitter |
|
||||||
- Youtube |
|
||||||
- Discord |
|
||||||
|
|
||||||
|
|
||||||
# Github |
|
||||||
|
|
||||||
## Github org rename |
|
||||||
- rename xgenecloud into nocodb |
|
||||||
|
|
||||||
## Create xgenecloud organisation |
|
||||||
- migrate repos : |
|
||||||
- xc-seed-rest, xc-seed-gql, xc-desktop-app |
|
||||||
- xgenecloud |
|
||||||
|
|
||||||
## Incorporate xmysql to nocodb |
|
||||||
- Move xmysql to nocodb |
|
||||||
- rename it to nocodb |
|
||||||
- make a commit mentioning xmysql will be nocodb |
|
||||||
- commit xgenecloud-ts into nocodb |
|
||||||
- create roadmap |
|
||||||
- remove/close issues |
|
||||||
- remove/close pull requests |
|
||||||
- headings |
|
@ -1,25 +1,12 @@ |
|||||||
# Google Oauth |
# Google Oauth |
||||||
|
|
||||||
|
<img width="1676" alt="1" src="https://user-images.githubusercontent.com/5435402/133758867-5976b85c-00c6-496f-874e-2a5cf6e0c882.png"> |
||||||
 |
<img width="579" alt="2" src="https://user-images.githubusercontent.com/5435402/133758880-bd8bf544-4366-4c22-8381-2237a8109424.png"> |
||||||
|
<img width="838" alt="3" src="https://user-images.githubusercontent.com/5435402/133758883-bab5ef0b-3b53-46c7-b602-1329f91e3c7f.png"> |
||||||
 |
<img width="1667" alt="4" src="https://user-images.githubusercontent.com/5435402/133758885-ac057a97-b3e5-480f-bcb8-9d08039a1f7c.png"> |
||||||
|
<img width="1208" alt="5" src="https://user-images.githubusercontent.com/5435402/133758888-606a0565-d76a-436d-9300-f0424425589e.png"> |
||||||
 |
<img width="1329" alt="6" src="https://user-images.githubusercontent.com/5435402/133758891-c9fdcc93-4450-4df8-a869-39e95d063467.png"> |
||||||
|
<img width="1125" alt="7" src="https://user-images.githubusercontent.com/5435402/133758894-b1dc612e-49e2-4956-b4e4-4a46cd37a4c4.png"> |
||||||
 |
<img width="1288" alt="8" src="https://user-images.githubusercontent.com/5435402/133758897-24feb534-279d-4d6c-b6e3-8b6827204896.png"> |
||||||
|
<img width="929" alt="9" src="https://user-images.githubusercontent.com/5435402/133758899-453299cb-3759-42b8-8904-136a9f86ac43.png"> |
||||||
 |
<img width="559" alt="10" src="https://user-images.githubusercontent.com/5435402/133758900-9979daf7-4db0-46c5-87da-6af5589e638a.png"> |
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
|
@ -1,22 +1,11 @@ |
|||||||
# AWS S3 |
# AWS S3 |
||||||
|
|
||||||
|
<img width="1674" alt="1" src="https://user-images.githubusercontent.com/5435402/133758975-3c56e136-1f0d-40d8-a835-4a039826bb09.png"> |
||||||
 |
<img width="849" alt="2" src="https://user-images.githubusercontent.com/5435402/133758989-9a3769c4-5bc1-4541-9871-0b99dc775a80.png"> |
||||||
|
<img width="815" alt="3" src="https://user-images.githubusercontent.com/5435402/133758993-29c0da2d-b216-4e35-84c3-14a51b1407cc.png"> |
||||||
 |
<img width="842" alt="4" src="https://user-images.githubusercontent.com/5435402/133758998-f9a56453-dacd-48ab-bd5b-50a50ebd6715.png"> |
||||||
|
<img width="1651" alt="5" src="https://user-images.githubusercontent.com/5435402/133759000-32a29de8-e00c-49a1-8410-1fb67ffa17f8.png"> |
||||||
 |
<img width="1671" alt="6" src="https://user-images.githubusercontent.com/5435402/133759001-be3fbf95-ce8a-43ee-b44f-cc35fd4ce021.png"> |
||||||
|
<img width="1670" alt="7" src="https://user-images.githubusercontent.com/5435402/133759003-fe8220a6-b9f8-402d-a7e2-8675fc2be9d8.png"> |
||||||
 |
<img width="1660" alt="8" src="https://user-images.githubusercontent.com/5435402/133759004-aa8f40be-56fa-431d-9ba7-d4d63a3fa8b5.png"> |
||||||
|
<img width="1523" alt="9" src="https://user-images.githubusercontent.com/5435402/133759009-24b2829c-35d0-4c3e-b144-74e934d383d6.png"> |
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
 |
|
||||||
|
@ -0,0 +1,23 @@ |
|||||||
|
--- |
||||||
|
title: 'API Tokens' |
||||||
|
description: 'API Tokens' |
||||||
|
position: 500 |
||||||
|
category: 'Developer Resources' |
||||||
|
menuTitle: 'API Tokens' |
||||||
|
--- |
||||||
|
|
||||||
|
|
||||||
|
## API Tokens |
||||||
|
NocoDB allows creating API tokens which allow it to be integrated seamlessly with 3rd party apps. |
||||||
|
|
||||||
|
### Creating API tokens |
||||||
|
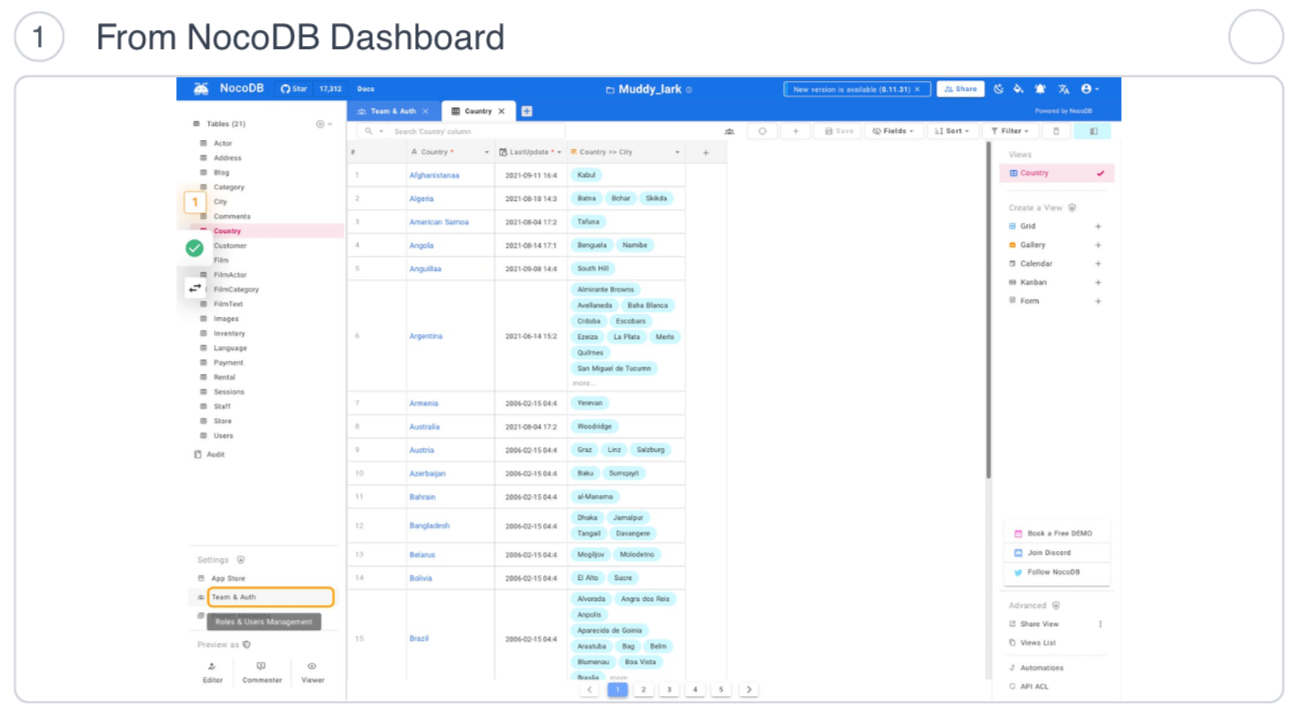 |
||||||
|
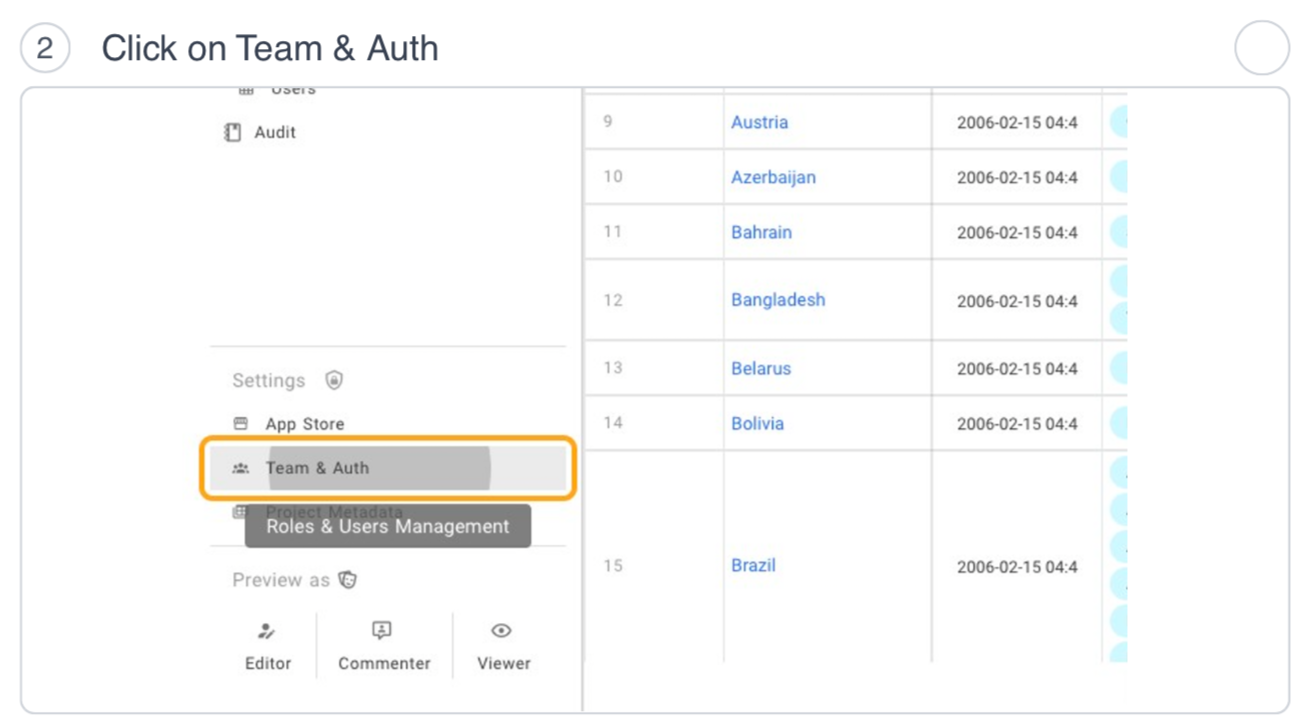 |
||||||
|
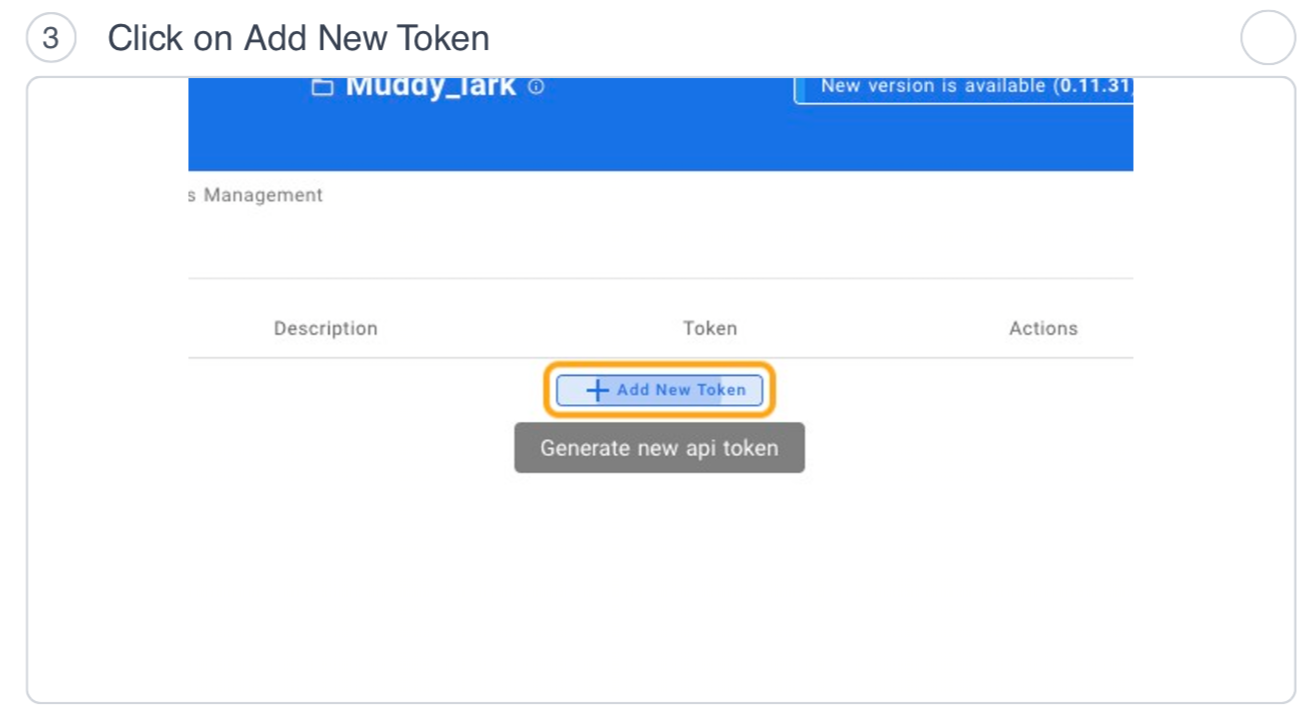 |
||||||
|
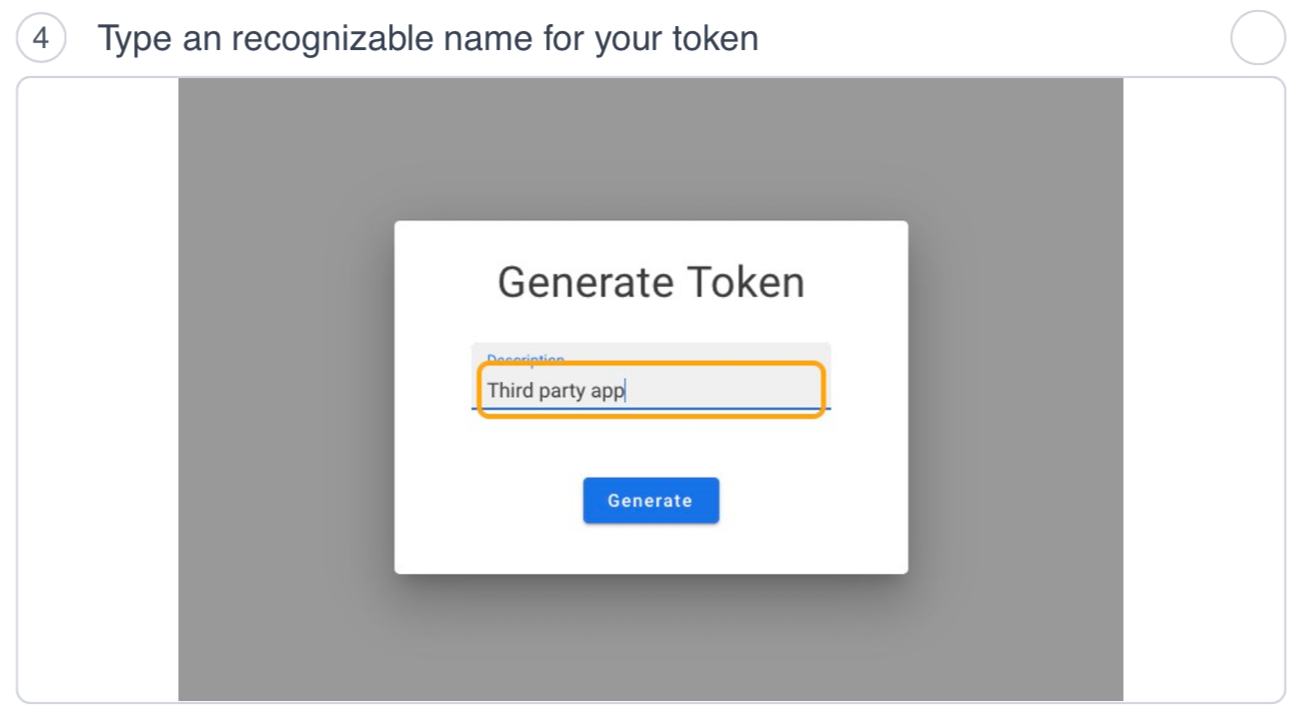 |
||||||
|
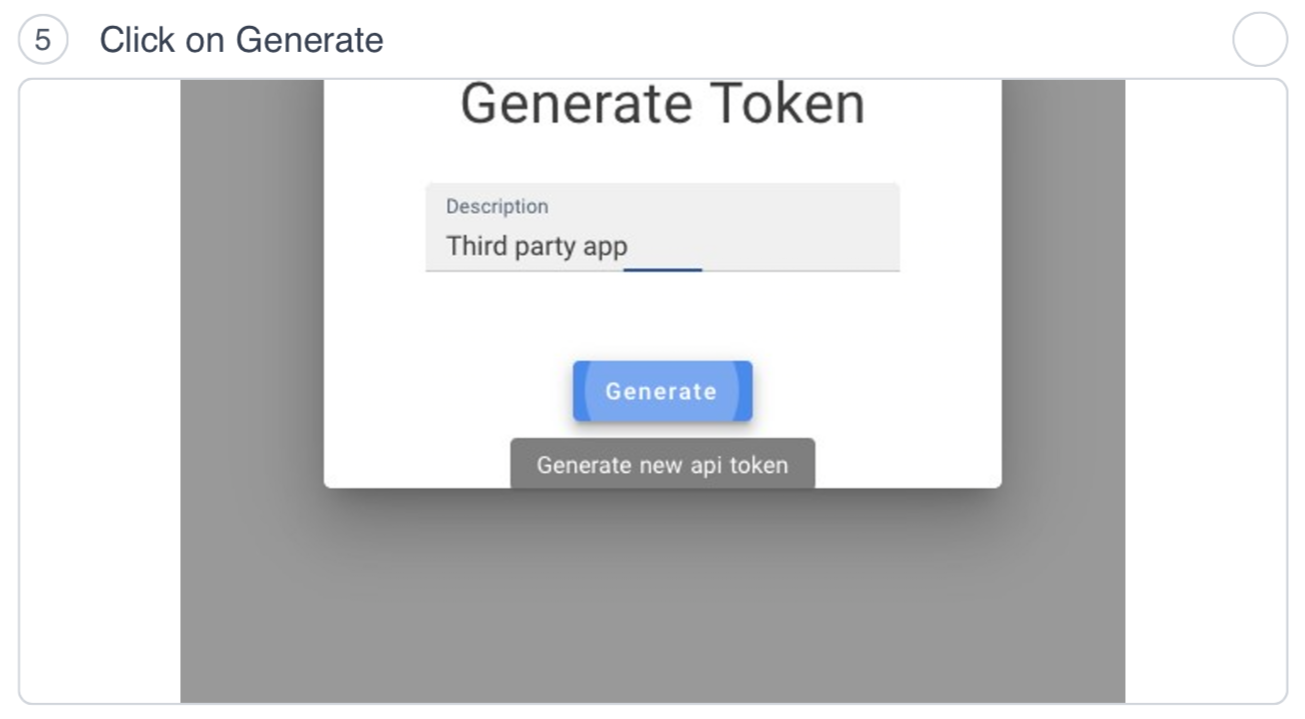 |
||||||
|
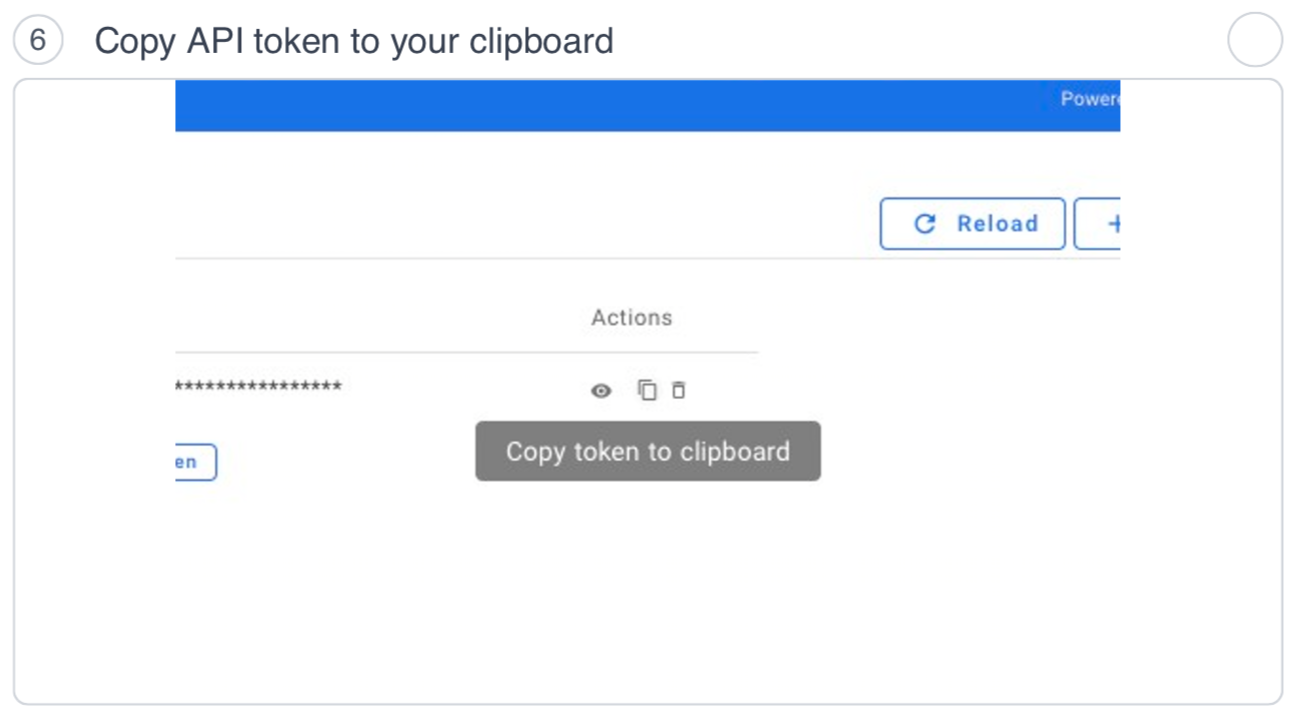 |
||||||
|
|
||||||
|
|
||||||
|
### Using API Tokens |
||||||
|
Invoke NocoDB APIs with ```xc-token``` header |
Before Width: | Height: | Size: 213 KiB |
Before Width: | Height: | Size: 113 KiB |
Before Width: | Height: | Size: 89 KiB |
Before Width: | Height: | Size: 117 KiB |
Before Width: | Height: | Size: 130 KiB |
Before Width: | Height: | Size: 188 KiB |
Before Width: | Height: | Size: 230 KiB |
Before Width: | Height: | Size: 130 KiB |
Before Width: | Height: | Size: 200 KiB |
Before Width: | Height: | Size: 205 KiB |
Before Width: | Height: | Size: 605 KiB |
Before Width: | Height: | Size: 130 KiB |
Before Width: | Height: | Size: 106 KiB |
Before Width: | Height: | Size: 1.2 MiB |
Before Width: | Height: | Size: 152 KiB |
Before Width: | Height: | Size: 206 KiB |
Before Width: | Height: | Size: 96 KiB |
Before Width: | Height: | Size: 373 KiB |
Before Width: | Height: | Size: 312 KiB |
Before Width: | Height: | Size: 238 KiB |
Before Width: | Height: | Size: 77 KiB |
Before Width: | Height: | Size: 892 KiB |
Before Width: | Height: | Size: 5.2 MiB |
Before Width: | Height: | Size: 294 KiB |
Before Width: | Height: | Size: 385 KiB |
Before Width: | Height: | Size: 304 KiB |
Before Width: | Height: | Size: 368 KiB |
Before Width: | Height: | Size: 48 KiB |
Before Width: | Height: | Size: 594 KiB |
Before Width: | Height: | Size: 300 KiB |
Before Width: | Height: | Size: 345 KiB |
Before Width: | Height: | Size: 415 KiB |
Before Width: | Height: | Size: 221 KiB |
Before Width: | Height: | Size: 277 KiB |
Before Width: | Height: | Size: 145 KiB |
Before Width: | Height: | Size: 104 KiB |
Before Width: | Height: | Size: 131 KiB |
Before Width: | Height: | Size: 158 KiB |
Before Width: | Height: | Size: 229 KiB |
Before Width: | Height: | Size: 277 KiB |
Before Width: | Height: | Size: 159 KiB |
Before Width: | Height: | Size: 303 KiB |
Before Width: | Height: | Size: 240 KiB |
Before Width: | Height: | Size: 545 KiB |
Before Width: | Height: | Size: 358 KiB |
Before Width: | Height: | Size: 404 KiB |
Before Width: | Height: | Size: 56 KiB |
Before Width: | Height: | Size: 697 KiB |
Before Width: | Height: | Size: 378 KiB |
Before Width: | Height: | Size: 428 KiB |
Before Width: | Height: | Size: 526 KiB |
Before Width: | Height: | Size: 261 KiB |
Before Width: | Height: | Size: 202 KiB |